In mobile app development, handling dates and times is a common requirement. Whether it’s scheduling appointments, setting reminders, or displaying time-sensitive information, having a reliable Date and Time Picker component is essential. In React Native, the DateTimePicker
component simplifies the process of selecting dates and times. In this blog, we’ll delve into when we might use the Date and Time Picker and how to implement a DateTimePicker component in your react native apps.
When Might You Use Date and Time Picker
Date and Time Pickers are invaluable when you need to capture specific points in time from the user. Common use cases include:
- Event Scheduling: Allow users to pick dates and times when they want to schedule an event, such as meetings, appointments, or reminders.
- Birthdays and Anniversaries: Collect users’ birthdates or important anniversaries to provide personalized experiences or send timely notifications.
- Booking Services: When users are booking services, like flights, hotels, or restaurants, they need to specify check-in and check-out dates and times.
- Countdowns: Develop countdown apps for upcoming events, product launches, or deadlines.
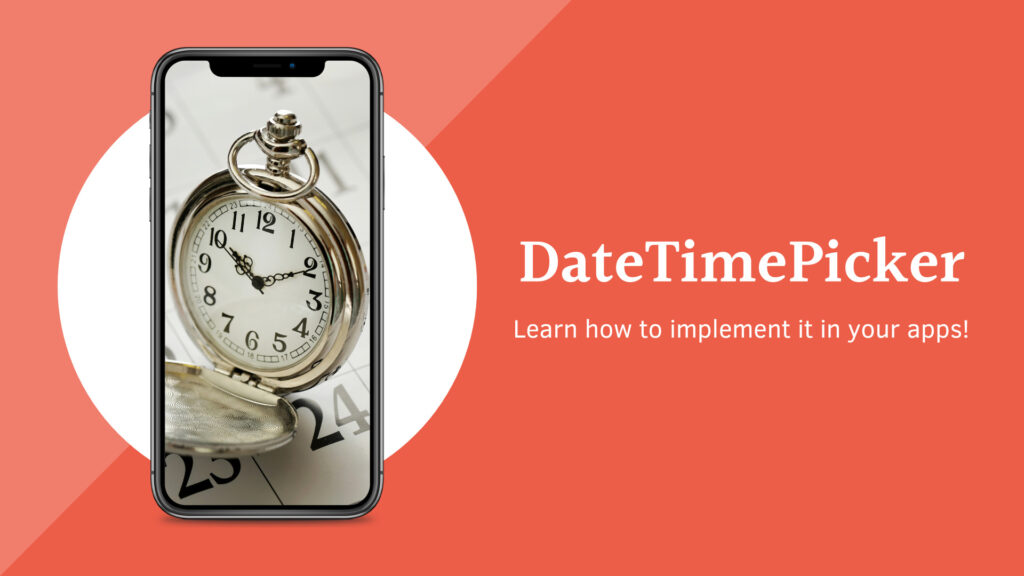
Understanding the Code
Let’s break down the provided React Native code for the Date and Time Picker:
import { StatusBar } from "expo-status-bar";
import { StyleSheet, View, Text } from "react-native";
import DateTimePicker from "@react-native-community/datetimepicker";
import { useState } from "react";
export default function App() {
const [date, setDate] = useState(new Date());
const onChange = (e, selectedDate) => {
setDate(selectedDate);
};
return (
<View style={styles.container}>
<DateTimePicker
value={date}
mode={"date"}
is24Hour={true}
onChange={onChange}
/>
<DateTimePicker
value={date}
mode={"time"}
is24Hour={true}
onChange={onChange}
/>
<Text>{date.toLocaleString()}</Text>
<StatusBar style="auto" />
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: "#fff",
alignItems: "center",
justifyContent: "center",
},
});
- Imports:
StatusBar
: This component controls the appearance of the status bar at the top of the screen.StyleSheet
: A utility for creating styles.
View
andText
: Basic components for structuring and displaying UI elements.DateTimePicker
: The core component for picking dates and times.useState
: A React Hook for managing component state.
- useState: The
useState
Hook is used to create a piece of state calleddate
. It’s initialized with the current date and time. If we call the setDate functions it updates the value of date, and triggers a re-render of components so the change in date is reflected on screen. - onChange: The
onChange
function is defined to update thedate
state whenever the user selects a date or time in theDateTimePicker
. - Rendering:
- Two
DateTimePicker
components are rendered, one for picking the date and another for picking the time. Both components are associated with thedate
state and share the sameonChange
function. In iOS you can specifydatetime
type and have only one picker, but this is not available in Android. For simplicity of code, I have chosen to display 2 DateTimePicker components. - A
Text
component displays the selected date and time using thetoLocaleString
method, which converts theDate
object to a human-readable string.
- Two
How It Works
When the app is loaded, the initial state for date
is set to the current date and time. As the user interacts with the DateTimePicker
components, the onChange
function is triggered. This function updates the date
state with the selected date or time. Since both DateTimePicker
components use the same state and onChange
function, they stay in sync.
The selected date and time are displayed below the DateTimePicker
components using the toLocaleString
method. This string provides a user-friendly representation of the chosen date and time.
Prefer to Learn by Watching?
If you are struggling to understand, the walkthrough tutorial of this is available on my MissCoding YouTube Channel! You can also find all this code on Github.
Conclusion
The Date and Time Picker component in React Native simplifies the process of capturing date and time information from users. By implementing the above code snippet and understanding its structure, you can create versatile apps that cater to scheduling, countdowns, and more. Harness the power of the Date and Time Picker to enhance user experiences and offer personalized functionalities in your React Native applications.