Facebook login is a useful feature for any app, it can improve the user experience by only requiring your users to login with an existing account and can also mean you can request permission to access some of your user’s facebook data. This can in turn be used to provide them additional features that integrate with data they have already provided to Facebook.
Today, we will be using react-native-fbsdk-next to configure our app for Facebook login. This package is a config plugin which means we don’t need to eject from Expo to use it, and can instead provide some config values in the app.json file. We then can build this as a standalone app and run it using expo-dev-client. For this package, we are unable to test in Expo go so I will also take you through how to build a standalone app using the eas build tools.
Getting Setup
To create your expo project, you will need to run the following command. You will replace expo-facebook-login-tutorial with your app slug.
npx create-expo-app expo-facebook-login-tutorial
You will also want to install expo-dev-client, as you will need this to run your standalone build. You can do this with the following command:
npx expo install expo-dev-client
Next you will want to update the app.json file with the bundleIdentifier for iOS and the package for Android, after doing so your app.json should look something like the following. Note: I have highlighted the package and bundleIdentifier so you can see the changes.
{
"expo": {
"name": "expo-facebook-login-tutorial",
"slug": "expo-facebook-login-tutorial",
"version": "1.0.0",
"orientation": "portrait",
"icon": "./assets/icon.png",
"userInterfaceStyle": "light",
"splash": {
"image": "./assets/splash.png",
"resizeMode": "contain",
"backgroundColor": "#ffffff"
},
"assetBundlePatterns": [
"**/*"
],
"ios": {
"bundleIdentifier": "com.misscoding.facebooklogin",
"supportsTablet": true
},
"android": {
"package": "com.misscoding.facebooklogin",
"adaptiveIcon": {
"foregroundImage": "./assets/adaptive-icon.png",
"backgroundColor": "#ffffff"
}
},
"web": {
"favicon": "./assets/favicon.png"
}
}
}
Next we will be eas to create the build configuration. If you don’t have it you can install the eas-cli it with the following command on line 1:
npm install -g eas-cli
eas login
eas build:configure
After we have the eas-cli tool installed, we can login if we haven’t previously done this and configure the build profile using eas build:configure
. This will generate an eas.json file, which I recommend adding a section to the development profile specifying you will be using the ios simulator. This will look as follows, with the additional lines highlighted:
{
"cli": {
"version": ">= 5.9.3"
},
"build": {
"development": {
"developmentClient": true,
"distribution": "internal",
"ios": {
"simulator": true
}
},
"preview": {
"distribution": "internal"
},
"production": {}
},
"submit": {
"production": {}
}
}
Next, we can generate the Android credentials which will be necessary for configuring the app on the Facebook developer site. You can follow on with the on screen questions accepting all the defaults, if you get stuck I have prepared a video walkthrough that you can refer to on my MissCoding YouTube Channel. If you find this content useful, please like and subscribe to help me grow and continue to contribute tutorials to the community. The command to generate the credentials is:
eas credentials
Keep track of the SHA1 generated above, we will use this when creating our app on the Facebook development site.
Creating Your Facebook App
The next step is to create our app: https://developers.facebook.com/apps/creation/
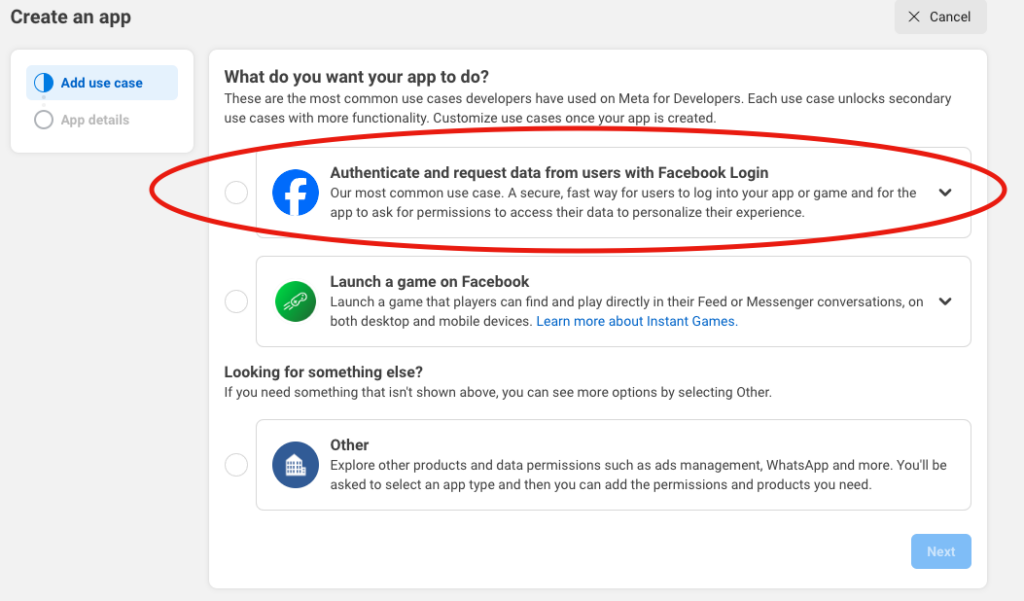
You will want to select the “Authenticate with FB” option. Then answer the on screen questions, and once those are completed click the Customize Facebook Login Button option. Here we can edit the permissions we are requesting, and also move to Quickstart which is where we want to go to configure our iOS and Android apps.
Let’s start with Android by selecting Android in the Quickstart menu. A reminder, that if you are stuck I have my video walkthrough available on YouTube. Once Android is selected, you can actually skip most of the steps as you are not implementing it natively. I would recommend adding a key hash and turning on single sign on (SSO). The key hash can be generated as follows:
- Convert SHA1 from eas credentials to Base64: https://base64.guru/converter/encode/hex
- Copy value into Add Your Development and Release Key Hashes
I would start by just adding my development key hashes, but once you are getting towards the release stage you will need to do the same for the release build.
In addition to the above, for Android, you will need to enter your package e.g. com.misscoding.facebooklogin is mine in this tutorial. And you will need to enter your MainActivity also, which for expo projects will be your package name with .MainActivity appended e.g. com.misscoding.facebooklogin.MainActivity.
You Android app should now be configured correctly for your Facebook app on the Facebook developer site, so now it’s time to configure iOS. For iOS, all you will need to do is enter your bundle identifier. Although, I also recommend turning on Single Sign On (SSO).
Once this is done, you can visit your app settings on Facebook. There are a couple of values that will be useful to you. The App ID which is in the basic settings, and the Client Token which is in the advanced settings. I will show you where to put these very shortly.
Configuring Your Config Plugin
Next, we will need to install react-native-fbsdk-next
and expo-tracking-transparency
. I believe expo-tracking-transparency
is only necessary if you are wanting to enable the delivery of personalized ads, so if you are setting this to false then I believe you can skip this step, and you would skip the associated code in following steps (it will be contained in the useEffect hook). The command to install these packages is as follows:
npx expo install react-native-fbsdk-next expo-tracking-transparency
After this, you will need to modify your app.json so it has the plugins section under expo (highlighted):
{
"expo": {
"name": "expo-facebook-login-tutorial",
"slug": "expo-facebook-login-tutorial",
"version": "1.0.0",
"orientation": "portrait",
"icon": "./assets/icon.png",
"userInterfaceStyle": "light",
"splash": {
"image": "./assets/splash.png",
"resizeMode": "contain",
"backgroundColor": "#ffffff"
},
"assetBundlePatterns": [
"**/*"
],
"ios": {
"bundleIdentifier": "com.misscoding.facebooklogin",
"supportsTablet": true
},
"android": {
"package": "com.misscoding.facebooklogin",
"adaptiveIcon": {
"foregroundImage": "./assets/adaptive-icon.png",
"backgroundColor": "#ffffff"
}
},
"web": {
"favicon": "./assets/favicon.png"
},
"extra": {
"eas": {
"projectId": "d25a6d12-94d8-41af-943d-083fc47af0b6"
}
},
"plugins": [
[
"react-native-fbsdk-next",
{
"appID": "651188800338806",
"clientToken": "3c38420d66a0c30403b1e367f148c9a3",
"displayName": "Login Tutorial",
"scheme": "fb651188800338806",
"advertiserIDCollectionEnabled": false,
"autoLogAppEventsEnabled": false,
"isAutoInitEnabled": true,
"iosUserTrackingPermission": "This identifier will be used to deliver personalized ads to you."
}
],
"expo-tracking-transparency"
]
}
}
The important things to change are the appID, clientToken, displayName and scheme. These should be backed on the Facebook app you just created and the scheme is just your appID prefixed by fb. The appID comes from the basic settings, and the client token comes from the advanced settings in the Facebook developer site.
Your expo react native app is now configured to connect to Facebook for authentication purposes, so now we just need a standalone build to test the logic!
Building a Standalone Build
I recommend building the standalone build before writing your code. There are a couple of reasons I suggest this:
- You can’t test Facebook Login on Expo Go, so a standalone build allows you to iteratively develop and get realtime feedback on your code
- Sometimes there are queues if you are on the free plan in particular and not building locally, so this means my standalone build will be available as early as possible for me
You can create your standalone build by running the following for iOS:
eas build -p ios --profile development
Or the following for Android:
eas build -p android --profile development
You can see that both pass the profile argument as development. This relates back to the build profiles in the eas.json file. As we have specified that we want to build for the iOS simulator, we will be able to install on the iOS simulator but not a physical device. My walkthrough on YouTube explores also building for a physical device using the preview build profile. However, it will require an active apple developer account.
EAS Build has some usage limitations when building on the Expo site, so you may opt to configure local building of your standalone apps. However, building on the Expo website is convenient and I typically am in no rush and don’t hit the limits.
The Code
Below I share the code I used to login with Facebook and also share a few other features that may be useful. For example, sharing a link, requesting data from the graph APIS and getting the access token. I will go into more detail about the code and what it does below.
import { StatusBar } from "expo-status-bar";
import { Button, StyleSheet, View } from "react-native";
import { useEffect } from "react";
import { requestTrackingPermissionsAsync } from "expo-tracking-transparency";
import {
AccessToken,
GraphRequest,
GraphRequestManager,
LoginButton,
Settings,
ShareDialog,
} from "react-native-fbsdk-next";
export default function App() {
useEffect(() => {
const requestTracking = async () => {
const { status } = await requestTrackingPermissionsAsync();
Settings.initializeSDK();
if (status === "granted") {
await Settings.setAdvertiserTrackingEnabled(true);
}
};
requestTracking();
}, []);
const getData = () => {
const infoRequest = new GraphRequest("/me", null, (error, result) => {
console.log(error || result);
});
new GraphRequestManager().addRequest(infoRequest).start();
};
const shareLink = async () => {
const content = {
contentType: "link",
contentUrl: "https://www.youtube.com/channel/UCwJWXcI12lhcorzG7Vrf2zw",
};
const canShow = await ShareDialog.canShow(content);
if (canShow) {
try {
const { isCancelled, postId } = await ShareDialog.show(content);
if (isCancelled) {
console.log("Share cancelled");
} else {
console.log("Share success with postId: " + postId);
}
} catch (e) {
console.log("Share fail with error: " + e);
}
}
};
return (
<View style={styles.container}>
<LoginButton
onLogoutFinished={() => console.log("Logged out")}
onLoginFinished={(error, data) => {
console.log(error || data);
AccessToken.getCurrentAccessToken().then((data) => console.log(data));
}}
/>
<Button title="Get Data" onPress={getData} />
<Button title="Share Link" onPress={shareLink} />
<StatusBar style="auto" />
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: "#fff",
alignItems: "center",
justifyContent: "center",
},
});
If you’ve come here purely for Facebook login, the code most of interest to you will be the LoginButton component I have added on line 61-67. It adds a button the user can click to login to Facebook. There are 2 properties I have specified against this function. We have onLogoutFinished which is called when the user has finished logging out, and for this I am just console logging the action. This is not particular useful and in reality you would probably want some kind of cleanup code to ensure your app reflects the fact that the user has logged out. We also have onLoginFinished, this is a property where you might configure your app state and you will either get an error e.g. user cancelled login or the user data. Once logged in, you may opt to request the AccessToken as shown on line 65. However, if you are just wanting to make Graph API calls with it, you are best to use the built in GraphRequestManager that I will explain shortly.
Let’s talk about lines 15-27 next. This code uses the useEffect hook, that means the callback passed to the useEffect hook is only called when the values described in the dependency array change. The dependency array is the second parameter, and as we have left this empty, useEffect will only call the callback once when the component first mounts. The callback itself requests tracking permissions and updates the settings of the Facebook SDK accordingly. The second arg is config and the final argument is a callback that will be called when the request is finished. Inside that callback you can do whatever you need with the data.
Next let’s talk about getting data from Facebook’s Graph API. On line 68, we add a button with the title “Get Data” that calls the getData
function when pressed. Inside the getData
function on lines 29-34, we create a GraphRequest. A GraphRequest takes in 3 arguments. The first is the endpoint, in this case we are using the /me
endpoint which requires very limited permissions. If we were requesting something requiring additional permissions, our app would need to specify these and it might require further review by Facebook to validate appropriate usage of the permissions.
Finally, we will talk about sharing content. On line 69, we add a button that has the title Share Link and calls the shareLink function. Note that while this is called shareLink in this instance, if we wanted to we could choose to share other content such as photos using the ShareDialog
that’s part of the SDK. The shareLink function is defined on lines 36-57. The first things we do is define the content we want to share, this should have a contentType and contentUrl in this instance. Then we check we can show the content. If we do, we try to show the content. If the user cancelled we store this value in the cancelled variable. If they are successful we get back the postId. We just console log the result, but you would likely want to give some visual feedback to the user. If there is an error, we catch it using the catch statement.
You can have Facebook login with sharing the link and getting data so if these don’t suit your use cases you are able to omit them.
Video Walkthrough
Everyone learns in different ways, for my more visual learned I have a video walkthrough that you can refer to on my MissCoding YouTube Channel.
Summary
Facebook Login can improve the usability and integration of your app with Facebook. Using this tutorial you should be able to utilise the basics of the react-native-fbsdk-next package. The code for this tutorial is available on GitHub.
If you’ve enjoyed this article, you may also enjoy my other tech blogs or my MissCoding YouTube channel! You can check out my code over on GitHub and follow me to get an early idea of what videos or articles will be coming next. Additionally, if you have enjoyed my content please support my continued content creation by donating via Stripe.